To explain tokens in C programming, let us look at an example. When we write a sentence in English, we use verbs, adverbs, and nouns to form a sentence. In order to construct a sentence, we need these basic things called building blocks.
Similarly, when you learn a new programming language, then you learn first about the basic building blocks that are Tokens because without basic building blocks we cannot understand the rest of the things.
Today we will learn in detail about What are tokens in C programming and what are the types of tokens in C programming?
What are Tokens?
- In the C programming language, tokens serve as the basic building blocks of a program.
- Tokens are the smallest units in a program.
- Creating a program in C without Token is similar to creating a sentence without using words.
- It is essential to use tokens when creating a C program.
- There are six types of tokens in the C language:
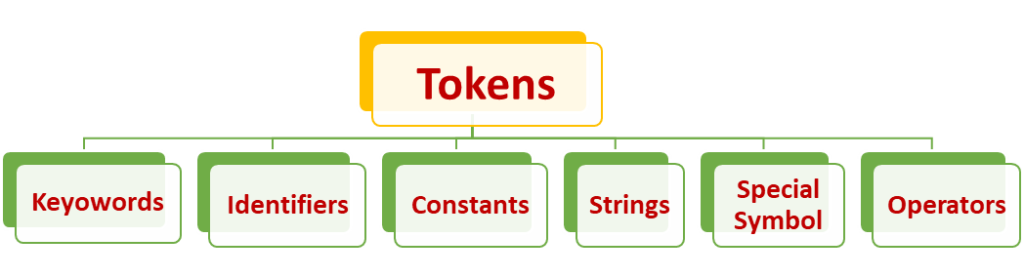
Keywords
- There is no way to change the meaning of any keyword.
- There can be no white spaces in keywords.
- The letters are always in lowercase.
- There is no way to use keywords as an identifier, variable name, constant name, etc.
- Each keyword has its own fixed memory.
- In C, there are 32 keywords. In the following table, you can see the keywords in C:
int | if | register | volatile | sizeof | for | const | goto |
float | else | extern | continue | return | while | unsigned | union |
double | switch | static | char | enum | do | signed | auto |
long | break | struct | void | typedef | default | short | case |
- The keyword is a pre-defined or reserved word in a C compiler. The compiler refers to keywords as referred names, so you cannot use them as variable names.
- It is impossible to change the meaning of keywords. They are therefore termed Reserve Words.
Identifiers
- Identifiers and modifiers are both the same thing.
- Users create these names by typing letters and digits.
- It is nothing more than the name of an element in a program that identifies it.
What are the rules for naming an Identifier?
If you want to use an identifier, you must follow these rules:
- The first character must be an alphabet.
- It must not contain white space.
- It should not begin with a number.
- The length of the Identifier depends on the compiler.
- An identifier should be easy to read, short, and meaningful.
- The only special character allowed is the underscore (_).
- You should not use keywords as an identifier.
- The upper case and lower case are distinct.
Example:
char name;
int emp_sal;
Where name and emp_sal are Identifiers.
Constants
- A constant is a fixed value that does not change during the execution of a program.
- Constants are also “Literals”.
- Constants in C have the following types:
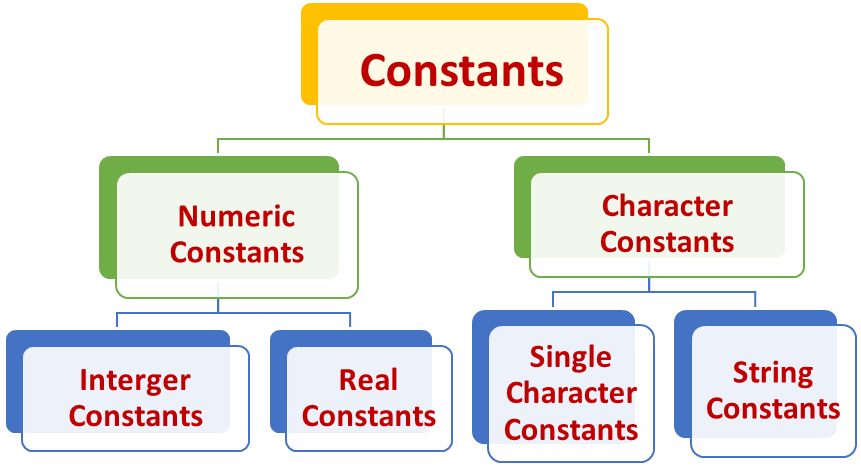
Numeric Constant
Numeric constants can either have or not have decimal points. It must have at least one digit.
Integer Constant
- The integer constants are whole numbers that don’t have fractional parts. Thus, Integer Constants consist of a sequence of digits.
- It can either be a positive or negative number.
- You may not use the comma (,) or a blank space ( ).
- There is a range of -32,768 to 32767 for the Integer Constant.
Example:
711
+713
-19801
20,000 /* Not valid, because comma (,) not allowed are Identifiers. */
Real Constant / Floating Point Constant
- There must be a decimal point in it.
- An exponential representation is also possible.
- There can also be a positive or negative exponent.
- The range is from -3.4e38 to 3.4e38.
Example:
+194.143
-416.41
Character Constant
It can be a single number, alphabet, or symbol encased in single or double quotation marks.
Single Character Constant
- It consists of a single character enclosed in single quotation marks (”).
- In a single character, there is a maximum length of 1.
- A Character Constant can be an alphabet, a digit, or another special symbol.
Example:
'D'
't'
'7'
String Constant
- Constants enclosed in double quotation marks (“”) are String Constants.
- Always a group of characters.
- As long as a single character is enclosed in double quotation marks, then it is treated as a String Constant, and not as a Character Constant.
Example:
"Hello World"
String
- The term “String” refers to a group of characters.
- C Strings are nothing, but an array of characters ended with a null character (‘\0’).
- There is a null character at the end of the String.
- There is no effect on the characters that follow the null character.
- Strings are stored as arrays of characters.
- An array consists of one location for each character in a string.
- You should always enclose strings in double quotes.
- It is necessary to include the string.h header file in order to access the string function.
- Strings can contain small letters, capital letters, numbers, and symbols.
Example:
"Hello World"
"Good Morning"
Special Symbol
Special symbols in C are symbols that have some special meaning and are not suitable for other uses.
- Braces {} – These opening and ending curly braces mark the start and end of a block of code containing more than one executable statement.
- Parentheses () – These special symbols are used to indicate function calls and function parameters.
- Brackets [] – A bracket serves as a reference to an array element. These indicate single and multi-dimensional subscripts.
- and (&) – Using this, we can find out the address of the variable.
- Asterisk (*) – This is used as a pointer to a variable.
- sizeof () – This gives the size of the variable.
- Comma (,) – The use of this operator is to separate multiple statements, as with parameters in a function call.
- Assignment Operator (=) – Using it, you can assign values
- Pre-Processor (#) – The pre-processor is a macro processor that is used automatically by the compiler to transform your program before actual compilation.
- Period (.) – For accessing union members.
Operator
- It is a symbol that represents an action, whether it is mathematical or non-mathematical.
- The purpose of this is to apply special operations to data.
- Operands are data items on which Operators act.
Unary Operator
In order to operate, this type of Operator requires only one operand or variable.
Example:
Increment / Decrement (++, --)
Binary Operator
It is mandatory to have at least two Operands for this Operator type.
Example:
= Equal Operator
+ Arithmetic Operator
Ternary Operator
Ternary operators are those that use three operands to perform an operation.
Example:
? :(Conditional Operator)
Conclusion
C programs consist of a variety of Tokens, and a token can be either a Keyword, Identifier, Constant, String, Special Symbol or Operator.